Brief Learning Notes about Context API in React
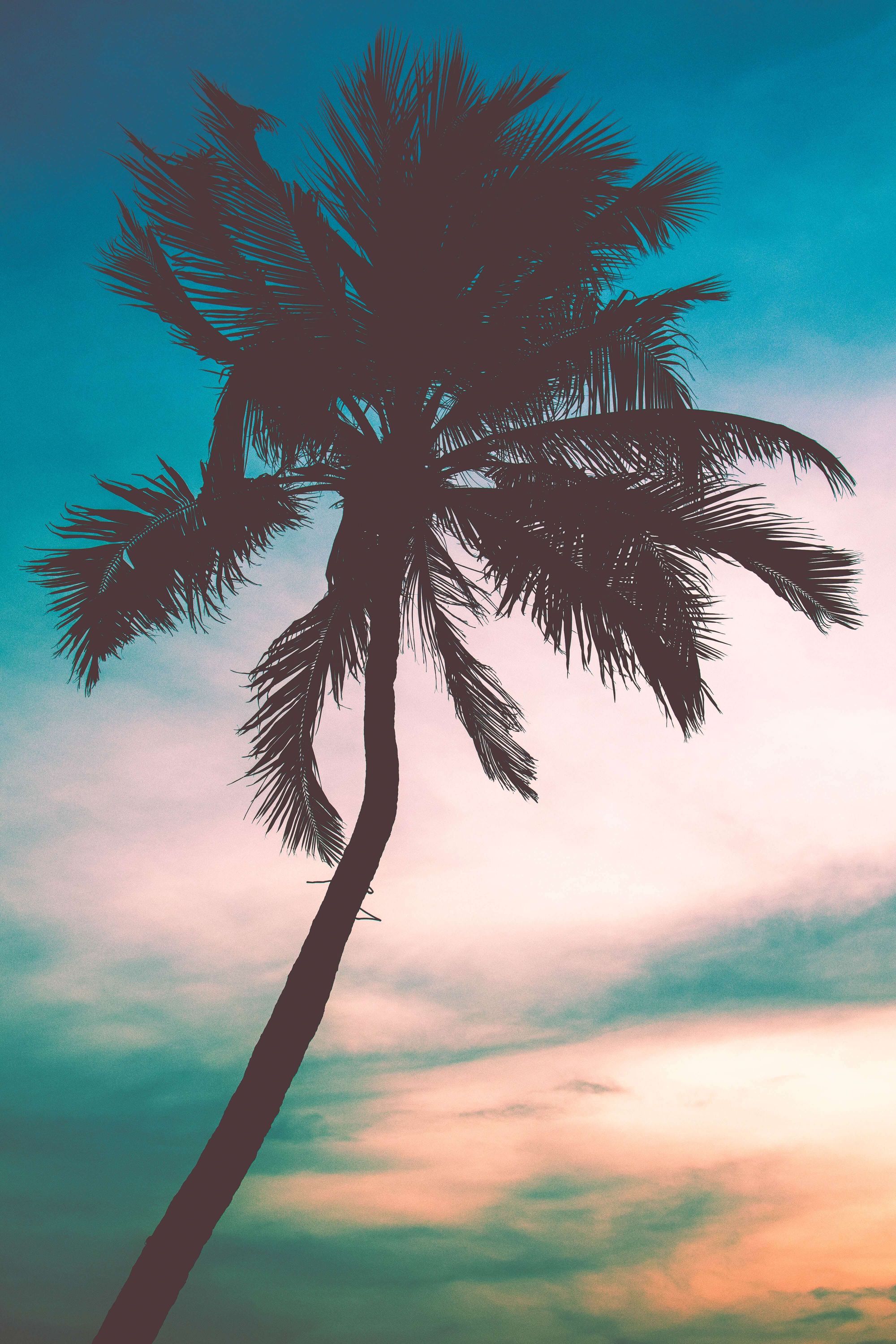
The Context API provides us with a global state. It allows us to access data without having to trickle the down props throughout the component tree.
What happens without a global state?
One of the major headaches of React is the lack of a global state. Since each component has its own state, accessible by only itself, the only way to pass data up and down the component tree is by lifting the state to a component higher up in the tree and pass down data as props from one parent to next child.
This could be troublesome for certain scenarios. From user settings to theme configurations, you have to pass the entire configuration down the component tree. This not only unnecessarily increases the props declared for every component but adding verbose logic to pass the props from the parent component to the child. The solution: a global state that is accessible throughout the component tree.
But doesn't Redux exist?
I am not well versed enough in both to talk about the pros and cons of Redux or make a comparison, but I can mention some similarities. For starters, they both have a similar approach to reading and writing the state. The state is read-only and we can write a reducer and let that manipulate our state. There is a dispatcher that matches the action and runs the corresponding reducer functions.
TL;DR of how Context API works
An overly simplified version of how the Context API work is:
There is a provider component. The provider will initialize it's own component state via the useReducer
hook, latching it onto the provider components (declared via createContext
) and then exporting these provider components for anyone to read the state or dispatch actions to run reducer functions (which will in-turn modify the state accordingly).
Any actual code to look at?
Yes, this time, I am going to share the demo I build to learn Context. This is a small todo-list demo that I wrote to learn Context from scratch. Take a look:
Additional Reads
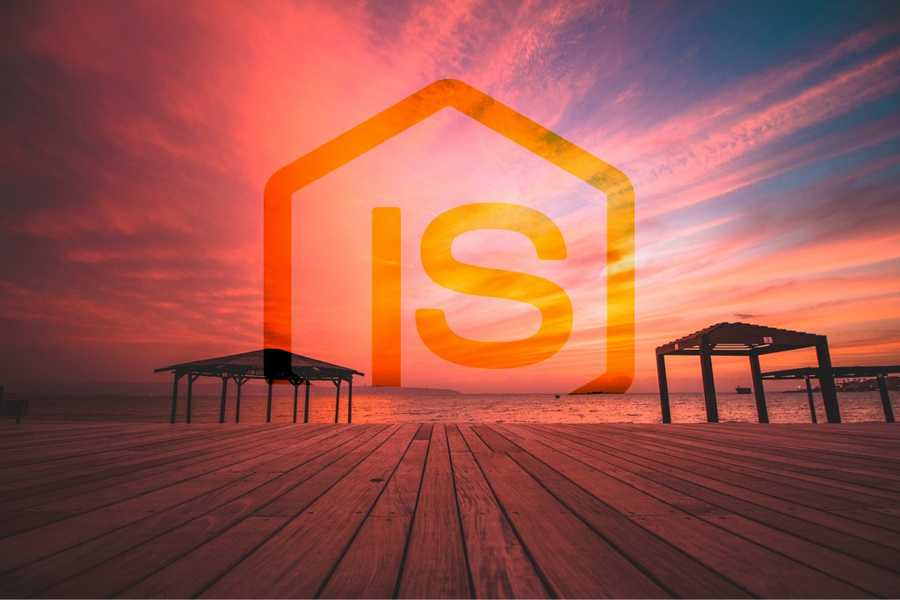
Videos
Photo by Phong Nguyen from Pexels