Writing a Pomodoro Timer with Vue.js - Part 1
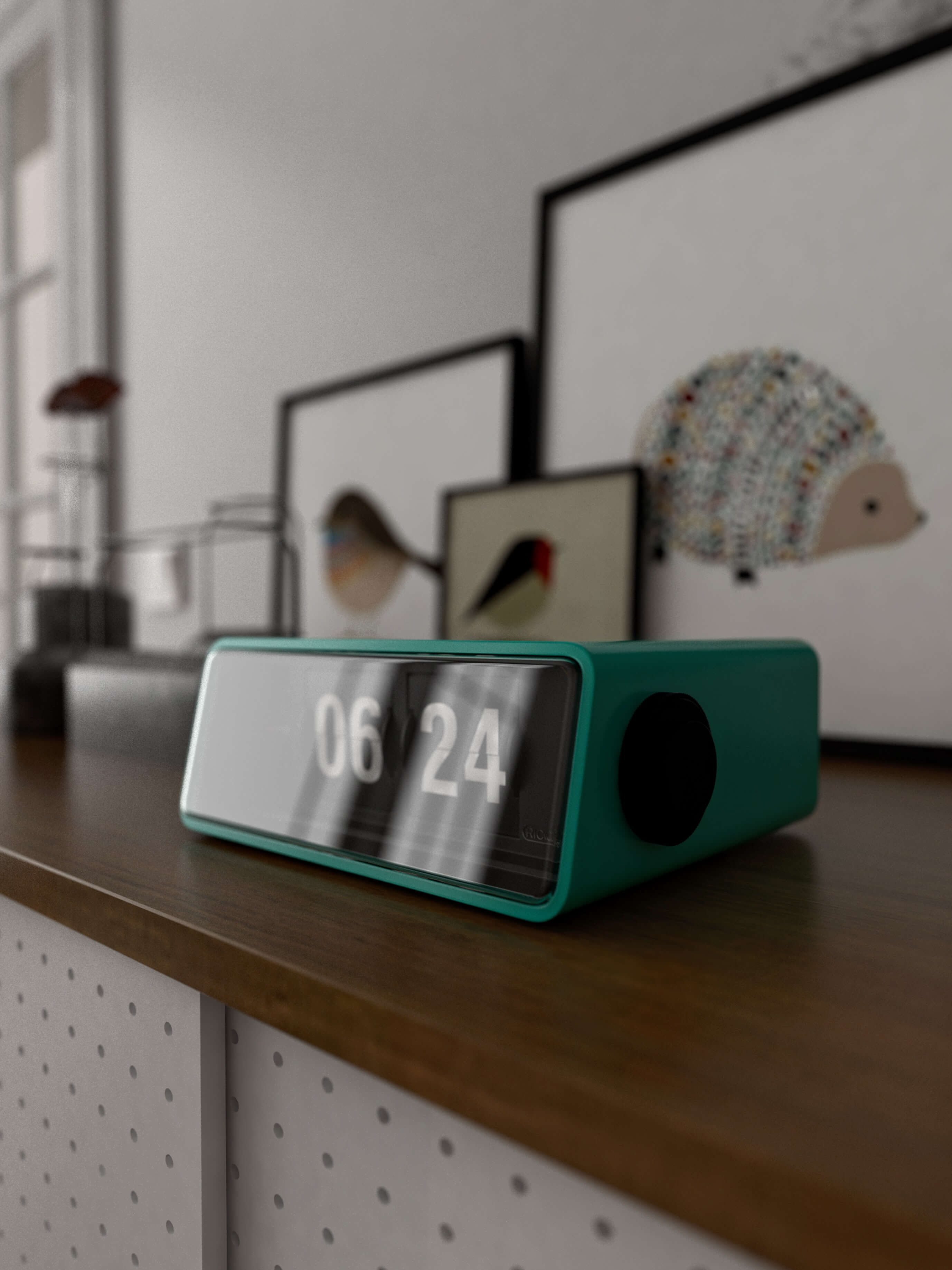
I wrote a simple Pomodoro timer based on Vue.js based on a project made by William Imoh. Check out the source on Github.
This is a simple 25 minute timer based on the Pomodoro Technique. The Pomodoro Technique is a time management method where work is done in roughly 25 minutes burst. I also hosted it on my github.io page as a submodule here.
In this part 1, I will briefly walk-through the Vue.js features I used, some things I learnt along the way and some JavaScript habits that I have.
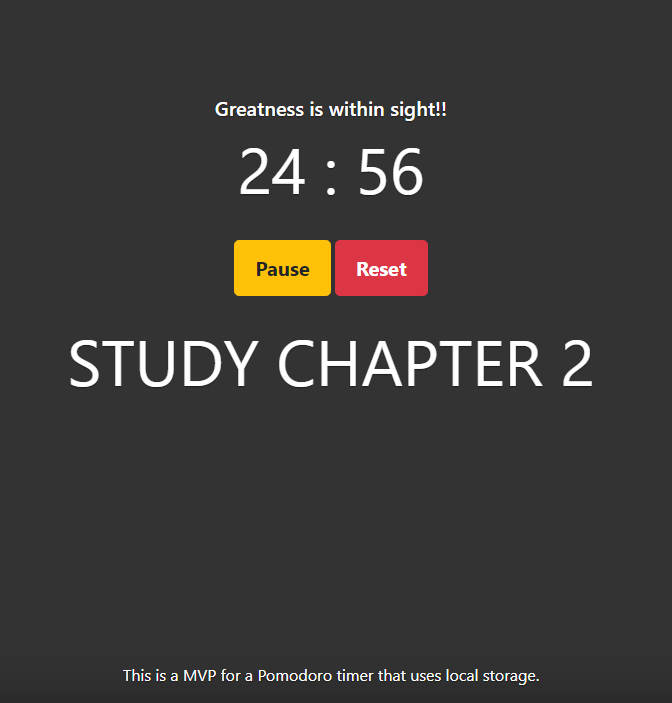
The core Vue.js stuff is done by William Imoh and posted on scotch.io. All I did was modify it, changed the naming conventions and made it slightly more dynamic. He had no problems with me writing about it when I DMed him on twitter. Check out his other works here.
Declaring the Vue.js Object
When declaring a new Vue object, I passed in an entire object with all of the data and functions it will need.
el
This represents the element the Vue object is trying to mount onto. This Stack Overflow answer explains it well.
https://stackoverflow.com/a/46596927/6622966
data
The Data object acts as an interface and allows the JSON data to be manipulated and/or bound elsewhere.
method
The method object act as an interface. It allows events that happened in the element to be mapped to Vue functions.
In the index.html file, we see a function assigned to the click action (similar to the onclick attribute on native HTML 5) <button id="start" ... @click="countingState">
And in the app.js file, we see the corresponding function countingState: function () { ... }
computed
This allows slightly complex functions to be extracted and computed separately. In app.js, I used countMinutes: function () { ... }
to represent the minutes and bound it with {{ countMinutes }}
on index.html.
Things I discovered
- I tried to put underscores in the
data
object to represent private access (it's just a naming convention), turns out you can't use underscores when dealing with JSON. - Vue.js is easy to pick up and start.
Some habits you might observe
Tabs or Spaces
I use 2 spaces instead of tabs. It doesn't matter what you use as long as you and your team are consistent. Most language compilers/runtimes/build-tools wouldn't care, we shouldn't either.
Underscores before function names
It is a naming convention that indicates the function is private. It doesn't do anything that prevents other functions from calling it elsewhere.
Double and Single quotes
Just a personal habit and naming convention, I normally use double quotes for HTML and single quotes for JavaScript. Both conventions work fine both ways, but it is a personal habit that I acquired.
CSS and JS tags
I always add my style sheets and scripting files at the bottom of the HTML file. In larger and more complex projects, some scripts might be loaded asynchronously and require the entire file to be parsed before loading the script. This is a small project and I'm not loading any scripts asynchronously, but I feel that doing this is a good habit.
In a future update
If my interest in this project doesn't die in a few days, I will add the following features:
- Allow the user to change the timing and the message
- Display a list of the completed task (Done)
- Use the localStorage API to store the settings and history mentioned above
- CI/CD process to tie up the loose ends for hosting (minify, concatenate files, etc)
Stock Photo by Oladimeji Ajegbile from Pexels