Rendering a Web Page Explained
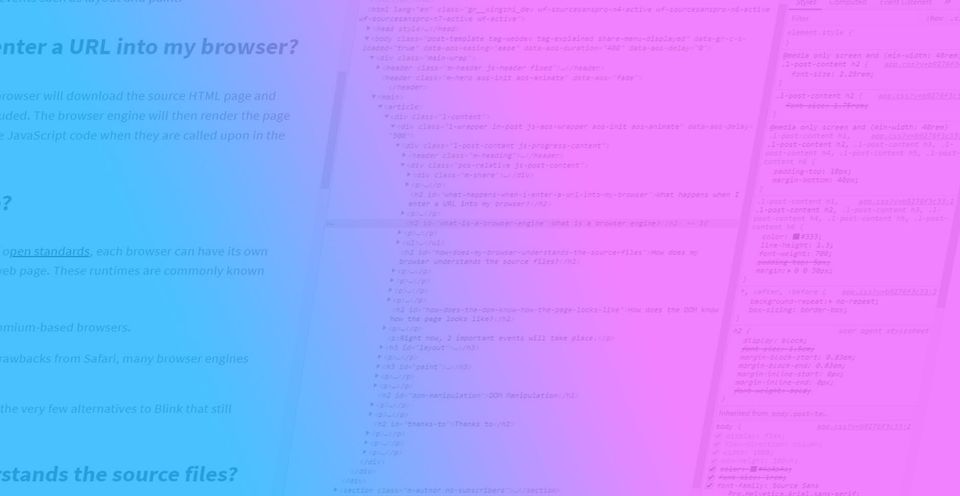
When you visit a website, your browser has to download the page as HTML or CSS or JavaScript files and render them into the web page you see. In this post, I will explain how the process works. I have been out of touch with these technologies due to my conscription service. As such I will not go into the details of DOM events such as layout and paint.
What happens when I enter a URL into my browser?
When a URL is entered into a browser, the browser will download the source HTML page and the corresponding CSS, JavaScript files included. The browser engine will then render the page in HTML, apply the CSS structure and run the JavaScript code when they are called upon in the HTML file.
What is a browser engine?
Since the web front end is definite with a set of open standards, each browser can have its own implementation of the runtime that renders a web page. These runtimes are commonly known as Browser Engines. They include
- Blink - Used by Chrome, Edge and most Chromium-based browsers.
- WebKit - Mainly used by Safari. Despite the drawbacks from Safari, many browser engines can have their origin tracked to WebKit.
- Gecko - Developed by Mozilla, Gecko is one of the very few alternatives to Blink that still provides many modern HTML 5 features.
How does my browser understands the source files?
The Document Object Model, DOM, is an interface of how your scripts can interact with the web page. It is a representation of the HTML code.
Upon receiving the source files the browser will translate them to their characters via their encoding type (basically, just reading the file as a .txt).
The parsing process happens next. Since most Markup files (including HTML) are strings enclosed in angled brackets, the browser can represent these enclosed tags as nodes. Each node having its own properties and attributes.
DOM Construction. The nodes are then represented as a tree structure, nested nodes brackets will be child nodes, etc. The DOM will be a representation of the markup and allows JavaScript code to interact and manipulate the elements nested within the DOM.
How does the DOM know how the page looks like?
In parallel, a similar process is happening on the CSS side of things. Once both the DOM and the CSSOM (CSS Object Model) are both constructed, they can be mapped together. The DOM will determine the structure of the nodes (who is a child of which parent node) and the CSSOM will determine the design of the web page (which nodes to hide based on their attributes, how to place each element beside each other, etc).
Right now, 2 important events will take place.
Layout
Layout, also commonly known as flow or reflow, is an event that happens after the DOM and CSSOM are mapped together. It determines which node appears on top of which and their respective dimensions.
Paint
Paint, also commonly known as repaint or paint setup, is an event that converts the entire tree structure and their layout into actual pixels and displays it to the user.
Every single time after a page is rendered, or the browser window is resized, these 2 events will be activated again in sequence. The page will have a new layout and a painted again to adjust to the new dimensions of the browser window. This enables responsive design where a web page will scale itself automatically when the browser window is adjusted or the device dimensions changed unexpectedly.
DOM Manipulation
This Mozilla Developer Network resource describes the basics of DOM manipulation. How to access data from the DOM, what kind of functions can be used to interact with the DOM.
https://developer.mozilla.org/en-US/docs/Web/API/Document_Object_Model/Introduction
This FreeCodeCamp resource explains how you can use some JavaScript browser APIs to interact with the DOM.
https://www.freecodecamp.org/news/whats-the-document-object-model-and-why-you-should-know-how-to-use-it-1a2d0bc5429d/
Thanks to
The majority of the content of this post are from my own experience and these resources:
Google's Web Fundamental Guides
https://developers.google.com/web/fundamentals/performance/critical-rendering-path/render-tree-construction
HTML5 Rocks Tutorial
https://www.html5rocks.com/en/tutorials/internals/howbrowserswork