React Functional VS Class Components - ReactJS
This is a short and opinionated comparison between the principles and motivations behind functional and class components in ReactJS. This is not a tutorial and should not be regarded as such.
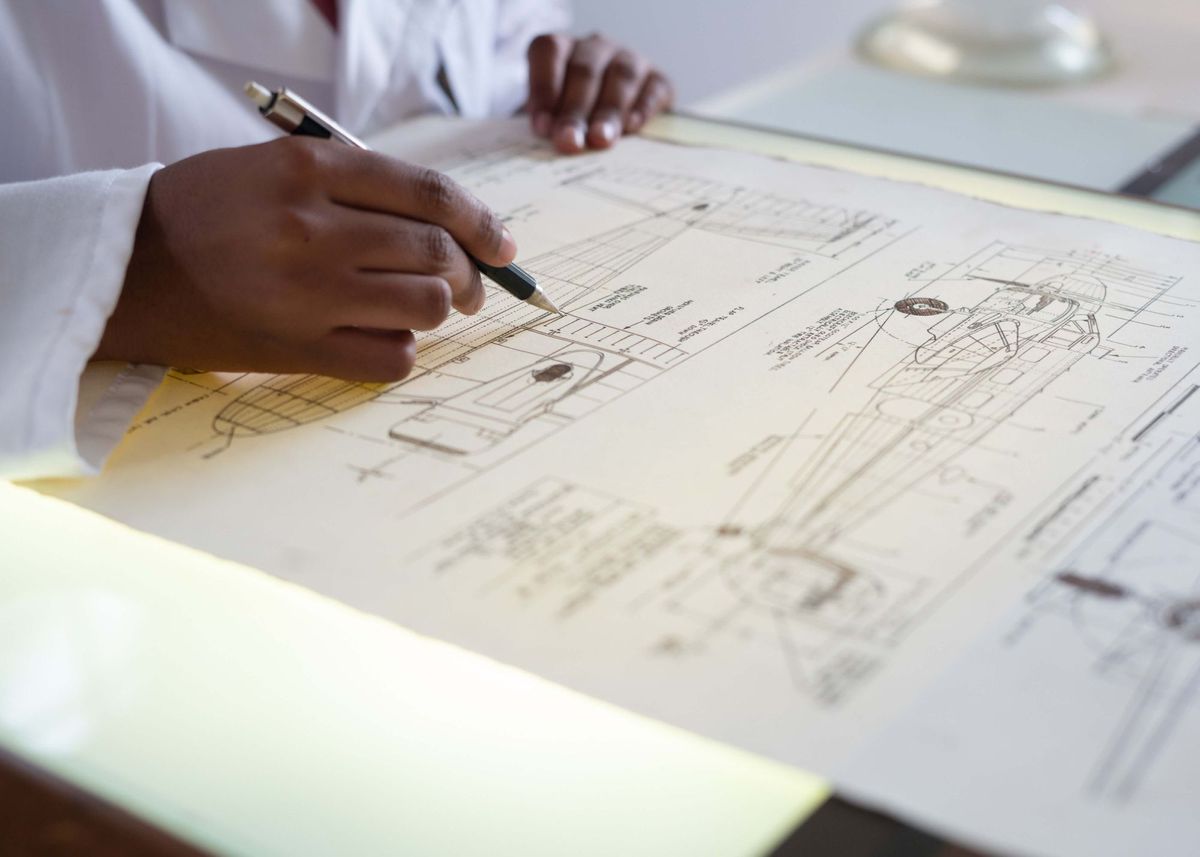
This is a short and opinionated comparison between the principles and motivations behind functional and class components in ReactJS. This is not a tutorial and should not be regarded as such.
What are those?
Class components were the de facto way to write components and they are easy to pick up. Similar to Android Fragments, each JSX class represents an entire UI widget, complete with their lifecycle events as functions within the class. It is incredibility easy to visualize the inner workings of a component.
Functional components, on the other hand, is a simple JavaScript function that returns a JSX element. You could do things like as manipulating state without using lifecycle events or running logic after a state change, but we will get into that later.
Why use Class Components?
I don't see a use case for class components anymore. Functional components are as capable as class components. When it comes to manipulating state, HOC pattern, accessing props, functional components can do all of these and offer many more features.
Why use Functional Components
React hooks. All of the functionality that we get from class components could be gained in some form or another with React hooks. Manipulating state, we can use the builtin useState
hook. Lifecycle events, we can use useEffect
. The main motivation for using React Hooks is to implement reusable behaviour without implementing new components in the hierarchy. You can read more about it here:
https://reactjs.org/docs/hooks-intro.html#motivation
Looking at this example, the business logic is abstracted into a JS function (this is a React Hook) and provides a very usable interface for the functional component to call. If this was to be implemented in a class component, we will need to create a HOC to implement the logic, thus complicating the hierarchy of our application and providing a syntax that is not only verbose but also less readable (for me at least). Not to mention the simpler DOM tree. With less wrapper components needed to implement logic, the DOM tree is more shallow and easier to debug.
So, which pattern should be use?
I prefer to use functional over class components any day. As someone who generally prefers functional programming patterns, I can see how powerful it is to spin up a JavaScript function to return a ReactJS component. And, without hooks, class components will certainly never be as powerful as their functional counterparts. Class components has easier to read syntax, being object oriented and all, but the appeal of React Hooks and functional programming patterns provided by functional components is hard to resist.
Additional Reading
- ReactJS has no plans to remove support for class components
https://reactjs.org/docs/hooks-intro.html#gradual-adoption-strategy - Deep diving into hooks
https://dev.to/dan_abramov/making-sense-of-react-hooks-2eib - Demoing React Hooks before it was a thing
https://youtu.be/dpw9EHDh2bM
Photo by Retha Ferguson from Pexels