Useful JavaScript Patterns
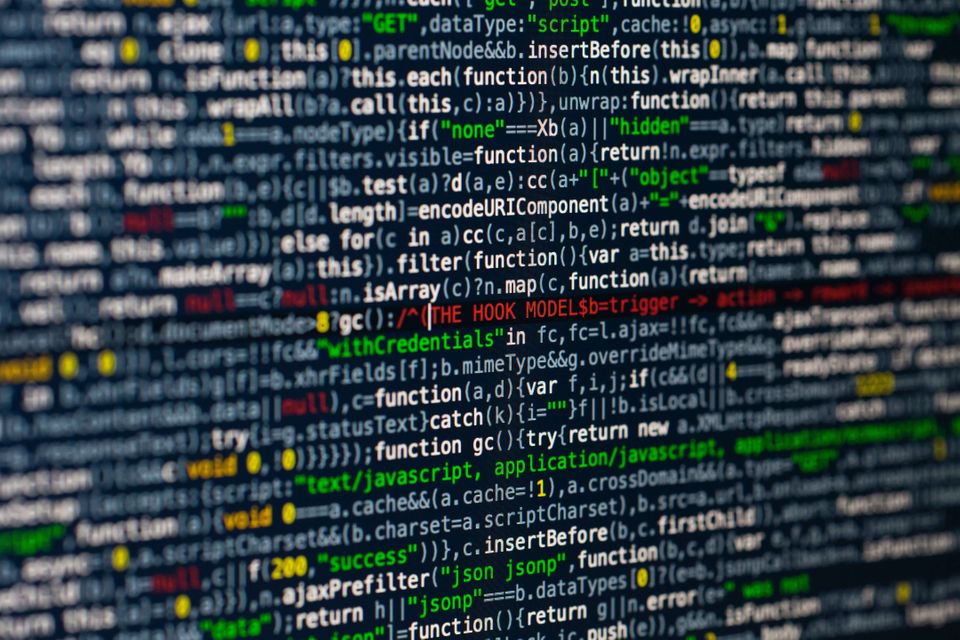
JavaScript might seems simple at first, but it has many wonderful and complex patterns. Let us explore what awesome stuff we can do with JavaScript.
Note: JavaScript is also known as EMCA Script and it is maintained by the ECMA International.
Arrow Functions
var me = {'name':'Lim'};
// Traditional function definitions
function traditionalFunction (input) {
input.name = 'xing';
}
traditionalFunction(me);
console.log(me.name); // prints xing
// Arrow functions in ES6 and beyond
me => me.name = 'zhi';
console.log(me.name); // prints xing
Syntax
The example above illustrates the syntax between a traditional function and arrow functions. An ES6 Arrow Function resembles a Lambda in Python.
Scope
While the scope of a traditional (or ES5) function will be inherited from its parent, the scope of an Arrow Function can only be called within its block. This is known as Lexical Scoping.
Why use Arrow Functions?
var countries =['Singapore', 'France'];
var countryLength = countries.map(function (country) {
return country.length;
});
console.log(countryLength) // prints [9,6]
var countryLengthArrow = countries.map(country => country.length);
console.log(countryLengthArrow) // prints [9,6]
With Arrow functions, you can create very short and readable code. You can use it everywhere you need a function expression, when you are using maps, callbacks, etc.
Immediately Invoked Function Expressions
Immediately Invoked Function Expression (IIFE) is a way to execute functions as soon as they are created without polluting the global namespace.
(function() {
/* your code here */
})()
or
(() => {
/* your code here */
})()
IIFE is mainly applied in situations where you will only need to use the expression once. Such as handing the DOMContentLoaded event or managing access control. For example:
// One time example
(() => window.alert('hello user'))(); // shows an alert to the user
// ==================================================
// Access Control example
var _websiteURL = (() => {
const url = "xingzhi.dev";
return url;
})()
console.log(_websiteURL); // prints xingzhi.dev
Comparison Operators and Primitives
JavaScript provides 2 types of comparison operator ===
and ==
.
===
also known as the Strict Equality Comparison or "strict equality".
==
also known as the Abstract Equality Comparison or "loose equality".
They both compare the value of 2 variable against each other. The main difference between the 2 is type conversion. When comparing between 2 variables, Abstract Equality Comparison will convert the type before comparing but Strict Equality will not. For example:
var fNumber = 1;
var fString = '1';
console.log(fNumber == fString); // true for Abstract Equality Comparison
console.log(fNumber === fString); // false for Strict Equality Comparison
However, consider this
var newStr = new String('9');
var str = '9';
console.log(newStr == str); // true
console.log(newStr === str); // false
To explain this behavior, we have to talk about Primitive. A primitive is a data that is not an object and has no methods. There are 7 primitive types in JavaScript: Number, BigInt, Boolean, String, Null, Undefined and Symbol. In the example above, we are declaring newStr
as an object instead of a string.
console.log(typeof str); // string
console.log(typeof newStr); // object
const priString = String('9');
console.log(typeof priString); // string
We can also see that priString
is a primitive string instead of an object. With the priString
example, we are declaring newStr
as an object using the new
keyword.
Additional Reading Materials
Arrow Functions
- https://medium.com/free-code-camp/learn-es6-the-dope-way-part-ii-arrow-functions-and-the-this-keyword-381ac7a32881#.59q9th108
- https://hackernoon.com/javascript-es6-arrow-functions-and-lexical-this-f2a3e2a5e8c4
Immediately Invoked Function Expressions
Primitive Data Types
- https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String#Distinction_between_string_primitives_and_String_objects
- https://codeburst.io/javascript-essentials-types-data-structures-3ac039f9877b
- https://medium.com/front-end-weekly/difference-between-string-primitives-and-string-object-d962b7ab8496
Photo by Markus Spiske temporausch.com from Pexels