How to write Promises in JavaScript
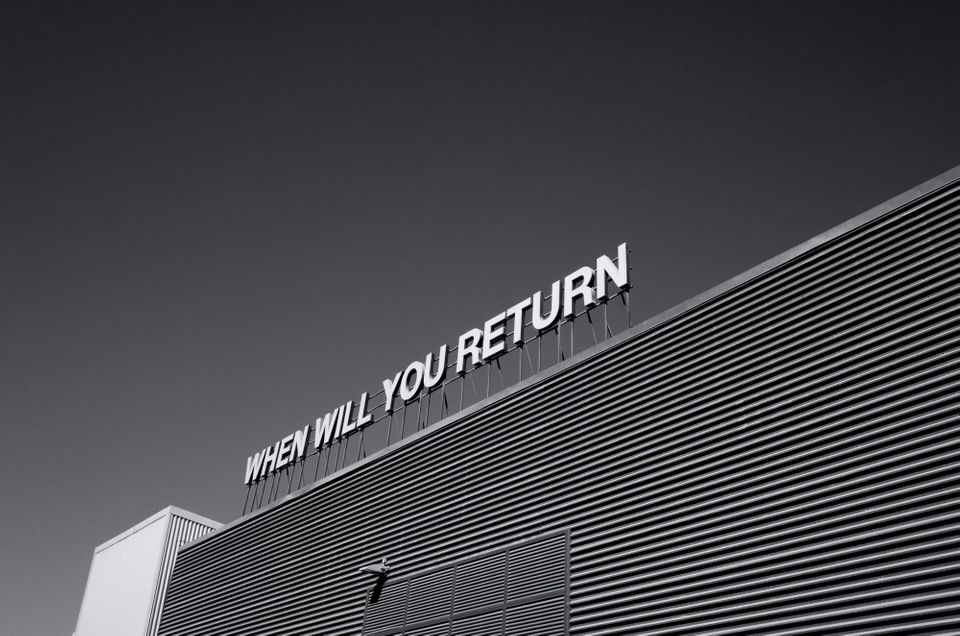
In Computer Science, a promise represents the eventual completion of a operation. In JavaScript, it allows asynchronous execution of functions without blocking the main thread.
I will be using typescript for most of the examples here. Think of it as JavaScript, but with strong types and a transpile stage that catches all my dumb errors before converting to JavaScript.
How does a promise looks like
Promises requires only one parameter, a function expression that is immediately executed (promiseExecution
). This function accepts 2 parameters, first parameter will be called when the promise is fulfilled (resolve
), the second will be called when the promise is rejected (reject
).
If you look at the logs printed, the function expression (promiseExecuted
) is immediately executed when the promise is declared.
Resolving a promise
Referring to the example above, a promise is fulfilled when the resolve
function is called. The caller can pass the resolve function with the promiseA.then
syntax.
The inverse applies for promiseA.reject
. A promise is rejected when the reject
function is called. This can be implemented with the promiseA.catch
syntax.
Promise Usage
One of the most prominent usage of Promises is the fetch
API. The fetch
API is a browser RESTful interface that allows browser-side JavaScript to do HTTP based requests.
fetch('http://example.com/movies.json')
.then(response => response.json())
.then(data => console.log(data));
What you see in this example is known as promise chaining. The fetch
function returns a promise that is resolved when the JSON data is returned from the RESTful endpoint. .next
returns a promise. That second promise will be resolved when the response from the first promise is parsed as a JSON object. Then, in the last function, the JSON data is printed to the console.
Async functions
Introduced in ES7, async functions always return a promise. Without the await
keyword, the promise will always resolve immediately after execution of its main thread.
With the typescript syntax, we can see that an async function always returns a promise.
References
- Promise - MDN
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise - Promise.then
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise/then - Promise Chaining
https://javascript.info/promise-chaining - Async Await
https://developer.mozilla.org/en-US/docs/Learn/JavaScript/Asynchronous/Async_await