Simple Web App with ReactJS
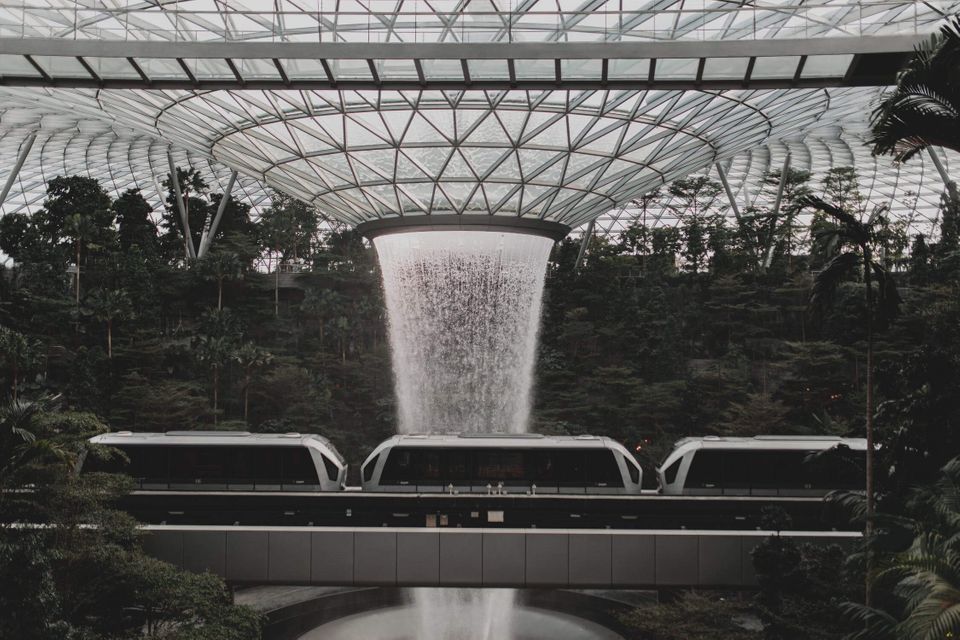
I wanted to learn ReactJS and I wanted to make a new browser tab page thingy. So, I made a new home page with ReactJS as I'm learning ReactJS (without Redux). What could go wrong?
Bfore we talk about ReactJS
Before we can talk about ReactJS, here are some HTML and JavaScript concepts you should know.
- Document Object Model (DOM) The DOM is an interface for manipulating your HTML elements. It represents all of your HTML elements as nodes and allows you to interact with them through JavaScript.
- Callback functions Sometimes in JavaScript, you can pass a function as a parameter. This function will execute when your logic is done executing. The function passed in is known as a callback.
- Events Events are messages that are sent out by HTML elements when something is happening. When your mouse clicks on a HTML button, it dispatches an event. When your DOM is done rendering a page, it dispatches an event.
- Event ListenersIn order to receive the event, you need to set a listener and a corresponding function act on the event.
- ES6 classesClasses are introduced in JavaScript with version ES2015. It is not an implementation object-oriented design, but rather a prettier version of the Object-Prototype pattern.
- Life cycle eventsLife cycle events are functions that run when certain events are triggered. On native Android, for example, you can define functions that will run when a screen activity is started, or when it is killed.
What is ReactJS?
ReactJS is a JavaScript based framework. Components make up the basic building blocks of the framework. They can contain JavaScript logic, HTML definition, CSS styling wrapped together in one ES6 class. You can define each component with the JSX syntax and each component have their own life cycle events.
React Components - The building blocks of ReactJS
A component is a widget that you can add to the DOM. It is defined as an ES6 class with certain life cycle events attached to it that you can handle. It contains a state
object that stores all private variables and a props
interface for you to interact with the component.
The entire component is packaged into one class
This is an example of a React component. It will take a string through props
and render it as a <h1>
tag with all upper case.
import React from 'react';
export default class Heading extends React.Component {
constructor(props) {
/* Pass the props interface to the parent class */
super(props);
/* Set state values to props */
this.state = props;
/* Checks if state.text exist and set it to 'title' if not */
if (!this.state.text) {
this.state.text = 'title';
}
/* Updating the 'text' value in the state object */
this.setState({
text: this.state.text.toUpperCase(),
}, function() {
console.log("Text is capitalized.")
});
}
render() {
return <h1> {
this.state.text
} </h1>;
}
componentDidMount() {
/* Print to console when the component is mounted */
console.log('Header mounted');
}
}
Defining the classexport default class Heading extends React.Component
We are defining Heading as a React Component by extending from the React.Component class. Heading will also be the default imported class when we import this file.
ConstructorWe are doing 3 things in the constructor. We are initializing the parent class with the default props arguments and initializing the state of Heading with props. Then we set the value of text within the state if it is not initialized with props. Finally, we capitalize the value of text.
setState
is asynchronous, meaning the browser will not wait for setState to finish running before it returns from the constructor.setState
does not override the entire state object. Instead, it will only override the values specified within the first parameter.setState
It accepts a call back function as a second parameter. In our case, we are printing a statement to the console when the state is updated.
props props
is an interface that exists in the "tag" of the component. It allows you to interact with the component when you write its tag. Continuing from the example above: <Heading text="This is a Heading"/>
state state
is the "private" variables of the component. It acts as a model to hold all of the component's data as shown above.
renderrender
is the compulsory lifecycle event. It generates the component it is called whenever a component has to be drawn.
Lifecycle eventsReactJS gives us a bunch of lifecycle events. In this example, I used the componentDidMount
event to print a message.
ReactJS VS the normal JavaScript way
Since ReactJS is based on a virtual DOM, there are many differences between it and a normal HTML web page with JavaScript logic.
Communications between widgetsTypically, JavaScript modules use events to communicate. It is simple, elegant, and the different modules do not have to be very coupled. Add it needs is an event listener at a higher level and a dispatcher at a lower level as shown above. ReactJS does it differently. Only the direct child can communicate with the parent and vice-versa through the props
interface mentioned above.
Manipulating ContentTraditionally, the easiest way to update HTML elements is to look for the element, and then changing it directly with JavaScript.
const element = document.queryselector(queryString)[0];
element.innerHTML = newContent;
With ReactJS, it is much simpler. Since every component has a state, we render contents from the state. And since the Virtual DOM binded to the state, every time we change the state means the DOM will change too!
render() { return <div>{ this.state.text }</div>; }
componentDidMount() { this.setState({ text: 'Hello world', });
How did I learn ReactJS
- Make up my mind that I want to learn ReactJS.
- Wait for a few days to make sure that I was sane when I made that decision.
- Google "ReactJS tutorial" and follow the first link.
- Decide that I want to use ReactJS to make a small application.
- Start the writing application.
- Start to think that ReactJS is too heavy for personal projects.
- Realize that I've a lot of gaps in my knowledge, keep reading.
- Realize that ReactJS isn't that bad. It is verbose, but also very enjoyable over time.
- Write a blog post to talk about it and link the application 👉🌐.
What's next?
- Learn other tools like state management with Redux.
- Figure out how other people do routing.
Things to read
- First result on Googlehttps://reactjs.org/tutorial/tutorial.html
- Life cycle eventshttps://projects.wojtekmaj.pl/react-lifecycle-methods-diagram/
- Bootstrap with ReactJS for easy styling and managing componentshttps://react-bootstrap.github.io/components/alerts
- Props vs Statehttps://flaviocopes.com/react-state-vs-props/
- React Concepts - Where to store and how to manage your states, etchttps://reactjs.org/docs/thinking-in-react.html